记录关于 Vue + element-ui 的系列问题
这里将记录自己工作或学习中关于Vue+element-ui
的系列问题,如果您有有更好的解决方法可以在下面进行评论喔~
DatePicker快捷选项
1 | import i18n from '@/lang' // 如果不需要 中英文则注释掉 |
在组件中直接引入,然后在data
声明变量使用就好。
1 | import rangePickerOptions from "@/utils/range-pick-opt"; |
template
代码
1 | <el-date-picker |
效果如下:
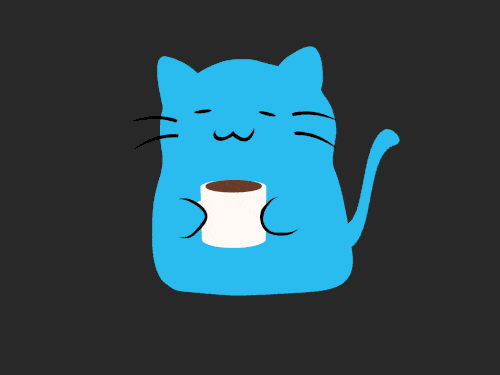
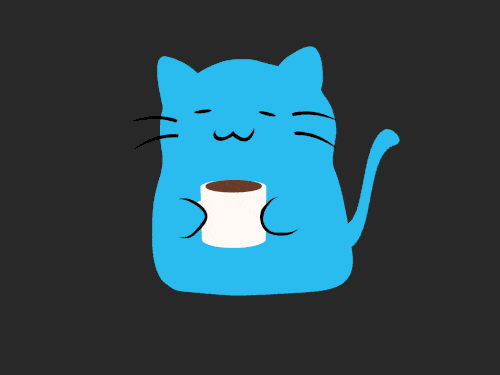
Select滚动加载 支持远程搜索
- 滚动加载数据
在入口文件main.js
中加入以下代码生成自定义指令1
2
3
4
5
6
7
8
9
10
11
12Vue.directive('loadmore', {
bind(el, binding) {
// 获取element-ui定义好的scroll盒子
const SELECTWRAP_DOM = el.querySelector('.el-select-dropdown .el-select-dropdown__wrap')
SELECTWRAP_DOM.addEventListener('scroll', function() {
const CONDITION = this.scrollHeight - this.scrollTop <= this.clientHeight
if (CONDITION) {
binding.value()
}
})
}
})template
代码1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20<el-select
v-model="form.tid"
clearable
filterable
remote
reserve-keyword
v-loadmore="loadMore"
:remote-method="remoteMethod"
>
<el-option
v-for="(item, index) in list"
:key="index"
:label="item.username"
:value="item.uid"
>
<div>
<span>自定义的内容</span>
</div>
</el-option>
</el-select>data
中需要声明的变量:1
2
3
4
5
6
7
8
9
10
11
12
13data(){
return{
status:'loadmore',
form:{
tid:0
},
list: [],
loadmorePage: {
pageNum: 1,
pageSize: 20,
},
}
}methods
中的方法:效果如下:1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29loadMore() {
if (this.status == "nomore") {
// 已经到最后一页了 return
return;
}
this.loadmorePage.pageNum += 1;
this.getList(); // 获取目标数据
}
async getList(value = "") {
const { Code, Data, Page } = await getApi({
Page: {
PageNum: this.loadmorePage.pageNum,
PageSize: this.loadmorePage.pageSize,
},
filter: value,
});
if (!Data || !Data.length) {
this.status = "nomore";
return;
}
// haxNextPage 是否有下一页
if (!Page || !Page.haxNextPage) {
this.status = "nomore";
this.list = this.list.concat(Data);
} else {
this.status = "loadmore";
this.list = this.list.concat(Data);
}
}往下滚动 加载第二页的数据往下滚动 加载第三页的数据往下滚动 继续 加载下一页的数据
直到加载完所有的数据 则 return - 远程搜索
methods
中的方法:效果如下:1
2
3
4
5
6remoteMethod(query) {
// query 搜索框输入的值
this.list = []; // 置空数据
this.loadmorePage.pageNum = 1; // 重置页码
this.getList(query); // 按 query 搜索
}
上传图片
template
代码:
1 | <el-form-item prop="srvimage" label="图片"> |
data
中需要声明的变量:
1 | data(){ |
1 | // Api接口 在页面引入即可 |
寻轮调用接口
给页面绑定一个ID,目的是为了 离开该页面后 不再轮询调用接口。
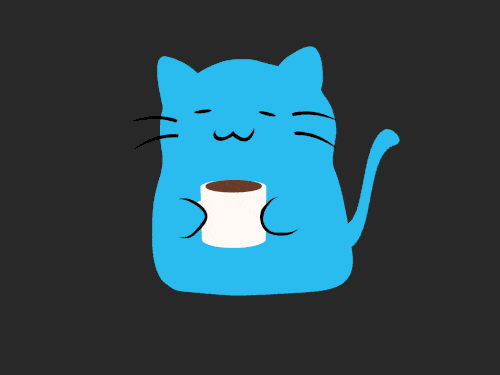
data
中需要声明的变量:
1 | data(){ |
methods
中的方法:
1 | getDataListSeconds() { |
本博客所有文章除特别声明外,均采用 CC BY-NC-SA 4.0 许可协议。转载请注明来自 唐志远!
评论