问题描述
使用echarts
的时候,多次加载会出现There is a chart instance already initialized on the dom.
这个黄色警告,大概意思就是dom
上已经初始化了一个图表实例。此警告信息不影响echarts
正常加载,但是有bug
不解决的话,心里痒的慌!
先说明一下,echarts
是用在了子组件的弹窗里,然后在父组件打开弹窗时调用echarts.init()
的初始化方法。第一次渲染正常,之后再打开弹窗控制台就会报There is a chart instance already initialized on the dom.
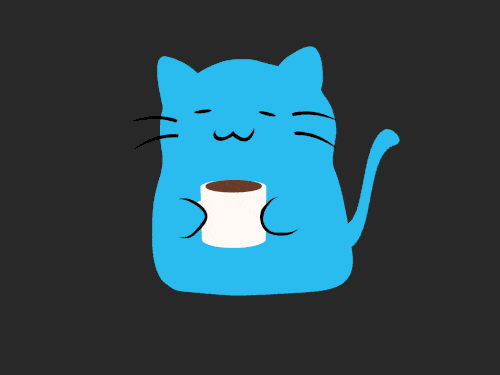
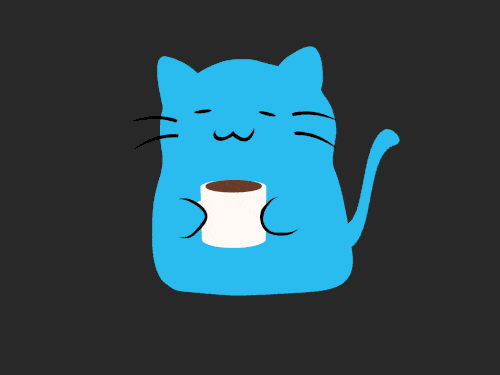
父组件中的代码:
1 2 3 4 5 6
| const taskDetailDom = ref() const open = ()=> { if (taskDetailDom.value) { taskDetailDom.value.initEchart() } }
|
如何造成的? 这里只区别了子组件的写法。
错误写法:
1 2 3 4 5 6 7 8 9 10 11 12 13 14
| <script setup lang='ts'> import echarts from "@/utils/custom/echart"
let tChart: Ref<HTMLDivElement | null> = ref(null) const initEchart = () => { const dom = tChart.value if (dom) { let myChart = echarts.init(dom) myChart.setOption(option) } } defineExpose({ initEchart })
|
关于import echarts from "@/utils/custom/echart"
此处中的代码(可参考官方示例)如下:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63
| import * as echarts from 'echarts/core'; import { BarChart, LineChart, PieChart } from 'echarts/charts'; import { LegendComponent, TitleComponent, TooltipComponent, GridComponent, DatasetComponent, TransformComponent } from 'echarts/components'; import { LabelLayout, UniversalTransition } from 'echarts/features'; import { CanvasRenderer } from 'echarts/renderers'; import type { BarSeriesOption, LineSeriesOption } from 'echarts/charts'; import type { LegendComponentOption, TitleComponentOption, TooltipComponentOption, GridComponentOption, DatasetComponentOption } from 'echarts/components'; import type { ComposeOption, } from 'echarts/core';
type ECOption = ComposeOption< | BarSeriesOption | LegendComponentOption | LineSeriesOption | TitleComponentOption | TooltipComponentOption | GridComponentOption | DatasetComponentOption >;
echarts.use([ LegendComponent, TitleComponent, TooltipComponent, GridComponent, DatasetComponent, TransformComponent, BarChart, LineChart, PieChart, LabelLayout, UniversalTransition, CanvasRenderer ]);
export default echarts;
|
解决方法
在方法最外层定义echarts dom
对象,然后echarts.init()
之前,判断dom
是否为空或未定义,如果已存在则调用dispose()
方法销毁,再初始化echarts.init()
。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17
| let tChart: Ref<HTMLDivElement | null> = ref(null) let myChart: any const initEchart = () => { const dom = tChart.value if (dom) { if (myChart != null && myChart != "" && myChart != undefined) { myChart.dispose(); } myChart = echarts.init(dom) myChart.setOption(option) } } defineExpose({ initEchart })
|